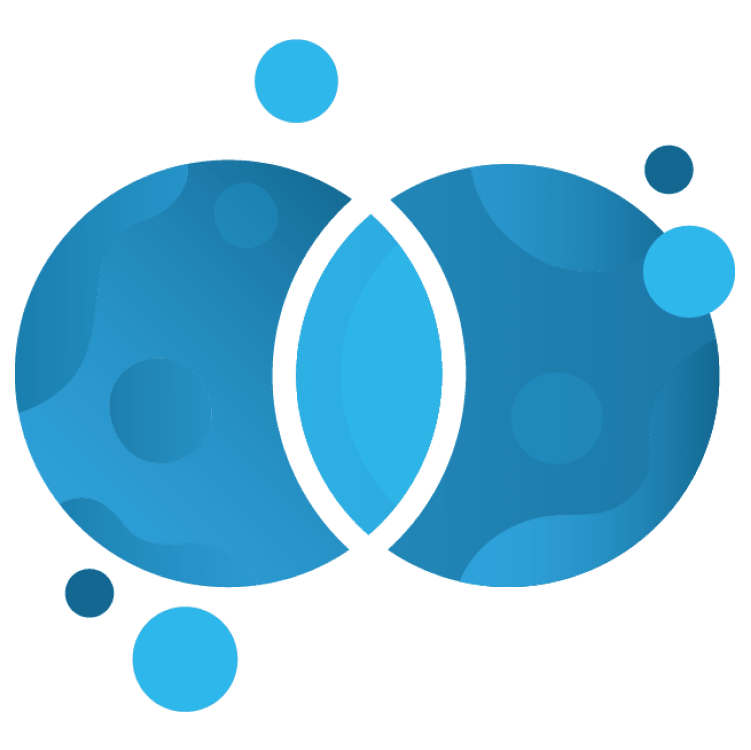
Dart Mixins Tutorial for Flutter: Getting Started
Learn about Flutter mixins, which help you implement some of the OOPs methodologies such as inheritance and abstraction in Dart. By Monikinderjit Singh.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Dart Mixins Tutorial for Flutter: Getting Started
15 mins
- Getting Started
- Exploring the Starter Project
- Setting up the Foundation for Mixins
- Recalling Inheritance and Composition
- Getting Into Mixins
- What Are Mixins?
- With, Implements and Extends
- Implementing Your First Mixin Class
- Integrating Multiple Mixins
- Testing Your Knowledge
- Understanding the Role of Hierarchy
- Why Use Mixins?
- Playing Safe With Mixins
- Where to Go From Here?
Object Oriented Programming plays a major role in the software development process. Many design patterns use the OOPs concept, so it’s important to know how to use different methods to make your application more scalable and debug easily. If you come from a tech background, you might have heard about inheritance, abstraction and other pillars of OOPs. Mixins help you implement some of these pillars.
In this section, you’ll learn about:
- Mixins
- How they’re different from inheritance
In the first section of this article, you’ll start by downloading the projects and required versions of Flutter and Android Studio. It’s time to start.
Getting Started
Download the starter project by clicking the Download Materials button at the top or bottom of the tutorial.
Open the starter project in VS Code or Android Studio. This tutorial uses VS Code, so you might need to change some instructions if you decide to go with Android Studio.
After opening the project, run Flutter Pub Get
in the terminal, to install the packages this project uses.
Exploring the Starter Project
Build and run the starter project. You’ll observe the following screen:
- When you build and run the project, the following screen will appear. It has two buttons.
- If you click either of these two buttons, nothing happens. Rather, if you open start_screen.dart, you find two
TODOs
where you’ll connect these screens. - In first_animation.dart and second_animation.dart file, the animations are already in place. The task is to create a common code base which you’ll implement using mixins.
Now, you’re all set to learn about mixins and start implementing your first mixin class.
Setting up the Foundation for Mixins
Before learning about mixins in Flutter, you first have to go quickly through the basics of OOPs. You’ll learn about inheritance and composition in the following section.
Recalling Inheritance and Composition
Definition of inheritance: Inheritance means inheriting/using the methods and functions of the parent class in the child class.
Definition of composition: Composition is the relation between different classes where the classes need not be the parent and child class. It represents a has a relation. The composition represents a strong relationship. It means if class A has Class B, then Class B can’t exist without class A.
How inheritance differs from composition: Inheritance defines the is a relationship where the child class is a type of parent class. Composition defines a part of relationship where the child is a part of the parent. Here’s an example:
Car is an automobile; it’s an example of inheritance.
Heart is part of human; it’s an example of composition.
Inheritance offers strong coupling, making it difficult to change or add functionality in the parent classes, whereas composition offers loose coupling. You might wonder why you would still use inheritance when you can use composition. The answer is that both have benefits and use cases, like how different fishing rods have their use cases.
Multiple inheritances in Flutter: Flutter doesn’t support multiple inheritances like some programming languages, such as C++. But there are ways to get the functionality of multiple inheritances in Flutter, which you’ll learn in the following sections.
Now, you have an image of the differences between inheritance and composition. In the next section, you’ll learn about mixins.
Getting Into Mixins
What Are Mixins?
Mixins are a language concept that allows to inject some code into a class. In Mixin programming, units of functionality are created in a class and then mixed in with other classes. A mixin class acts as the parent class (but is not a parent class), containing the desired functionality.
The following mixin code snippet will help you understand this better:
Without using Mixin Class:
class WithoutMixinClass{
void run(){
print("Hey, without mixin class's method running!!");
}
}
class OtherClass extends WithoutMixinClass{
}
void main(){
OtherClass obj=OtherClass();
obj.run();
}
In the snippet above, you inherit WithoutMixinClass
, which is inherited in OtherClass
using the extends
keyword. So the OtherClass
is the child class of WithoutMixinClass
.
Using Mixin Class:
mixin MixinClass{
void run(){
print("Hey, mixin class's method is running!!");
}
}
class OtherClass with MixinClass{}
void main(){
OtherClass otherClassObj=OtherClass();
otherClassObj.run();
}
In the snippet above, you use MixinClass
in OtherClass
using the with
keyword. The OtherClass
isn’t the child class. You need to use the with
keyword to implement mixins.
Are you getting confused between the with, implements and extends keywords available in Dart? No worries. The following section will clarify your understanding.
With, Implements and Extends
If you have some basic experience with Flutter, you might have already used implements
and extends
. In this section, you’ll learn the differences between with
, implements
and extends
.
Extends keyword:
- Used to connect abstract parent classes with child classes.
- Methods of abstract classes need not be overridden in child classes. This means if there are 10 methods in the abstract class, the child class need not have to override all 10 methods.
- Only one class can be extended by a child class (Dart does not allow multiple inheritance)
Implements keyword:
- Used to connect interface parent classes with other classes. Because Dart does not have any interface keyword, the classes are used to create interfaces.
- Methods for interface classes need to be overridden in child classes. This means if there are 10 methods in the interface, the child class needs to override all 10 methods.
- Multiple interface classes can be implemented.
With keyword:
- Used to associate mixin classes with other classes.
- Every method of the mixin class is imported to the other class.
- Multiple mixin classes can be used with the same class.
With the basics clear, you’re ready to implement the first mixin class in the Flutter project. You’ll learn about this in the next section.
Implementing Your First Mixin Class
At this stage, you have enough knowledge to start implementing your first mixin class. If you have gone through the project structure, open base_screen.dart. Copy and paste the following code snippet in place of //1.TODO: create mixin class
.
//1
mixin BasicPage<Page extends BaseScreen> on BaseState<Page> {
@override
Widget build(BuildContext context) {
return Scaffold(
floatingActionButton: fab(),
appBar: AppBar(
title: Text(screenName()),
),
body: Container(
child: body(),
color: Colors.black,
));
}
//2
Widget fab();
Widget body();
}
Here’s how this code works.
- To declare mixins in Flutter, you need to use the
mixin
keyword.BasicPage
is the name of mixin, which has an object type ofPage
that extends theBaseScreen
abstract class. - Here, you declare functions that, if needed, you’ll implement in the child classes.
This just set up your custom mixin class. The project application requires multiple mixins because you’ll also use SingleTickerProviderStateMixin
, which creates tickers in Flutter.
In the next section, you’ll connect mixins and observe them working.