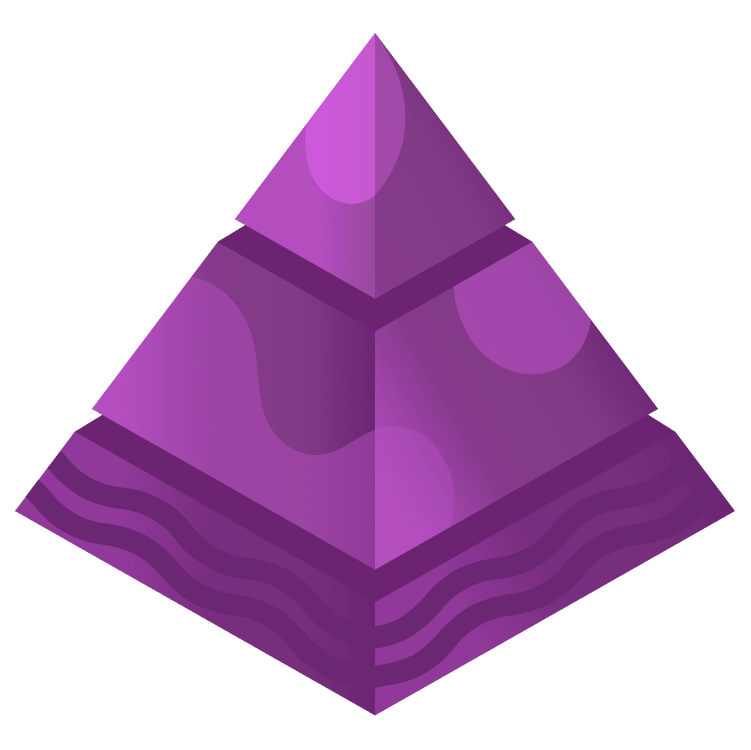
Introduction To Unity Unit Testing
Learn all about the Unity Test Framework and how to set up Unit Tests in your Unity projects. By Ben MacKinnon.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Introduction To Unity Unit Testing
35 mins
- What is a Unit Test?
- Looking at Example Unit Tests
- Getting Started
- Getting Started with the Unity Test Framework
- Setting Up Test Folders
- What’s in a Test Suite?
- Setting Up the Test Assembly and the Test Suite
- Writing Your First Unit Test
- Passing Tests
- Adding Tests to the Test Suite
- Setting Up and Tearing Down Phases
- Testing Game Over and Laser Fire
- Asserting Laser Movement
- Ensuring Lasers Destroy Asteroids
- To Test or Not To Test
- Unit Testing Pros
- Unit Testing Cons
- Testing that Destroying Asteroids Raises the Score
- Code Coverage
- Where to Go From Here?
Testing is a part of game development that’s often not given enough attention — especially in smaller studios without the resources for a dedicated testing team. Fortunately, as a developer, you can proactively use unit tests to help produce a top-quality project. In this tutorial, you’ll learn the following about unit testing in Unity:
- What is a unit test?
- What value do unit tests offer?
- Pros and cons to writing unit tests.
- How to import and use the Unity Test Framework.
- Writing and running unit tests that pass.
- Using Code Coverage reports to analyze how thorough your test cases are.
What is a Unit Test?
Before diving into code, it’s important to have a solid understanding of what unit testing is.
A unit test tests a single “unit” of code. Exactly what makes up a “unit” varies, but the important thing to keep in mind is that a unit test should test one thing at a time. Usually, this is a single method in your game logic.
You should design a unit test to validate that a small, logical snippet of code performs as you expect it to in a specific scenario. This might be hard to grasp before you’ve written any unit tests, so consider this example:
You wrote a method that allows the user to input a name. You wrote the method so there are no numbers allowed in the name, and the name must be 10 characters or less. Your method intercepts each keystroke and adds that character to the name
field as shown below:
public string name = ""
public void UpdateNameWithCharacter(char: character)
{
// 1
if (!Char.IsLetter(char))
{
return;
}
// 2
if (name.Length > 10)
{
return;
}
// 3
name += character;
}
Here’s what’s going on in the code above:
- If the character is not a letter, this code exits the function early and doesn’t add the character to the string.
- If the length of the name is 10 characters or more, it prevents the user from adding another character.
- Once those two cases pass, the code adds the character to the end of the name.
This method is testable because it does a “unit” of work. Unit tests enforce the method’s logic.
Looking at Example Unit Tests
How would you write unit tests for the UpdateNameWithCharacter
method?
Before you get started implementing these unit tests, think carefully about what the tests do and name them accordingly.
Take a look at the sample method names below. The names make it clear what’s being tested:
UpdateNameDoesntAllowCharacterIfNameIsTenOrMoreCharactersInLength
UpdateNameAllowsLettersToBeAddedToName
UpdateNameDoesntAllowNonLettersToBeAddedToName
From these test method names, you can see what you’re testing and that the “unit” of work performed by UpdateNameWithCharacter
is doing what it should. These test names might seem long and specific, but they can be helpful for you, or any other developer that comes along later, should any of the tests fail. A descriptive name will reveal the potential issue far quicker than having to dive into the code and check all the parameters around the test case.
Every unit test you write makes up part of a test suite. A test suite houses all unit tests related to a logical grouping of functionality. If any individual test in a test suite fails, the entire test suite fails.
Getting Started
Download the starter project by clicking the Download Materials link at the top or bottom of the tutorial. Open the Game scene in Assets / Scenes.
Click Play to start Crashteroids, and then click the Start Game button. Use the left and right arrow keys to move the spaceship left and right.
Press the space bar to fire a laser. If a laser hits an asteroid, the score will increase by one. If an asteroid hits the ship, the ship will explode and it’s game over (with the option to start again).
Play for a while, and then allow the ship to get hit by an asteroid to see that game over triggers.
Getting Started with the Unity Test Framework
Now that you know how the game works, it’s time to write unit tests to ensure everything behaves as it should. This way, if you (or someone else) decide to update the game, you can be confident in knowing the update didn’t break anything that was working before.
Before you write tests, make sure the Unity Test Framework is added to the project. Go to Window ▸ Package Manager and select Unity Registry from the drop-down. Scroll down the list to find the Test Framework package and install or update.
With the package installed, you can now open Unity’s Test Runner. The Test Runner lets you run tests and see if they pass. To open the Unity Test Runner, select Window ▸ General ▸ Test Runner.
After the Test Runner opens as a new window, you can make life easier by clicking the Test Runner window and dragging it next to one of your other windows to dock it.
Setting Up Test Folders
Unity Test Framework is the unit testing feature provided by Unity — but it utilizes the open source library NUnit. As you get more serious about writing unit tests, you should consider reading the docs on NUnit to learn more. For now, everything you need to know will be covered here.
In order to run tests, you first need to create a test folder to hold your test classes.
In the Project window, select the Assets folder. Look at the Test Runner window and make sure PlayMode is selected.
Click the button that says Create PlayMode Test Assembly Folder. You’ll see a new folder appear just under the Assets folder. The default name Tests is fine, so press Enter to finalize the name.
There are two different tabs inside the Test Runner:
The PlayMode tab is for tests that will run while in Play mode, as if you were playing the game in real time. The EditMode tests will run outside of Play mode, which is great for testing things like custom Inspector behaviors.
For this tutorial, you’ll focus on PlayMode tests, but feel free to experiment with EditMode testing once you feel ready.