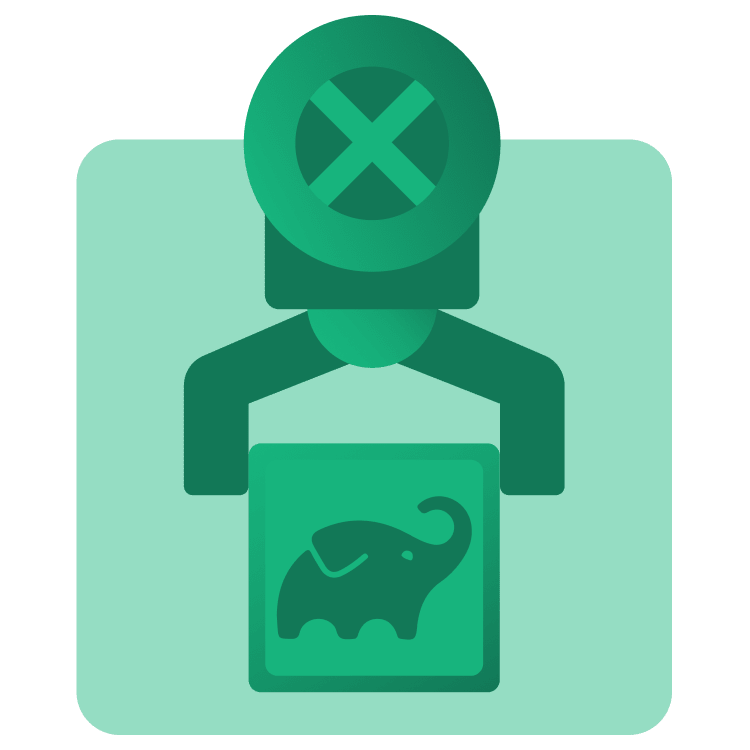
Gradle Tutorial for Android: Getting Started – Part 1
In this Gradle Build Script tutorial, you’ll learn the basic syntax in build.gradle files generated by Android Studio. You’ll also learn about gradlew tasks, different dependency management techniques, and how to add a new dependency to your app. By Ricardo Costeira.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Gradle Tutorial for Android: Getting Started – Part 1
35 mins
- What is Gradle?
- Getting Started
- Exploring the Project-Level Files
- Moving on to Module-level Files
- Graduating to the Settings Files
- Using New(er) Gradle Configurations
- Mastering the Build: Gradle Commands
- Defining gradlew
- Executing gradlew Tasks
- gradlew assemble
- gradlew lint
- Managing Dependencies
- Using the ext Property
- Using buildSrc
- Using Version Catalogs
- Including Picasso
- Configuring Gradle Dependency
- Where to Go From Here?
Graduating to the Settings Files
The next file will be quite short in comparison to the previous. Move to the settings.gradle file in the root directory. Here’s what you’ll see:
include ':app'
Here, you define all of your project’s modules by name. You have only one module – app. In a large, multi-module project, this file can have a much longer list.
Using New(er) Gradle Configurations
This is the structure that you typically find in older Android projects. It’s still perfectly valid, but if you create a new project on Android Studio, you’ll notice that the configuration is different for settings.gradle and project-level build.gradle.
You’ll now migrate Socializify’s configuration to the newer structure. Start by opening the project-level build.gradle. Delete everything, and paste the following:
plugins {
id "com.android.application" version "8.2.2" apply false
id "org.jetbrains.kotlin.android" version "1.9.20" apply false
}
tasks.register('clean', Delete) {
delete rootProject.buildDir
}
The plugins
block replaces the previous dependencies
block. Here, you declare the plugins you want to apply to the project. As you don’t want to apply these plugins at the root level, you write them with apply false
at the end. You can then apply them to your modules, through the corresponding module-level Gradle file, by using a plugins {}
block without the version (i.e., as you already did before).
The repositories
block now belongs in settings.gradle. Open the file and add this code above the include ":app"
line:
// 1
pluginManagement {
repositories {
google()
mavenCentral()
gradlePluginPortal()
}
}
// 2
dependencyResolutionManagement {
repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS)
repositories {
google()
mavenCentral()
gradlePluginPortal()
}
}
In this code:
- You define a
pluginManagement
block where you declare the repositories for plugins. Gradle uses these to resolve plugins like the ones you just added in build.gradle. You can also use this block for things like defining plugins with specific versions, adding aresolutionStrategy
block to detail how to resolve plugins, etc. - Define a
dependencyResolutionManagement
block where you declare the repositories for dependencies. Gradle uses these to resolve library dependencies you include in the project. You also define a RepositoriesMode. By default, any repositories you declare in a project’s build.gradle will override the ones you declare in settings.gradle. By settingrepositoriesMode
toFAIL_ON_PROJECT_REPOS
, you effectively force the build to fail if any repositories are declared outside this file.
The Android build system suggests having repositories declarations in settings.gradle since Gradle 7. It provides a centralized repository declaration available to all projects, instead of repeating repository declarations across all sub-projects. Plus, Gradle needs to resolve all plugins in order to configure the build. Since settings.gradle is executed before build.gradle, it makes sense to have the plugin management there so that it gets dealt with sooner.
After these changes, your project is out of sync. To fix this, press the Sync Now call to action at the top of the editor:
Don’t be afraid to re-sync your project. It takes a short while until the sync is completed, but it takes longer when you have more dependencies and more code in your project.
The project should build successfully. The .gradle files are now equivalent to the .gradle.kts files, with the appropriate changes in syntax. You’ll soon get to touch those as well, but your next step is to learn how to use Gradle.
Mastering the Build: Gradle Commands
To execute Gradle commands, use the command line or Android Studio. It’s better to start with the command line to get better acquainted with what’s going on. For this, you’ll use gradlew.
Defining gradlew
gradlew is the Gradle Wrapper. You don’t need to worry about installating Gradle on your computer – the wrapper will do that for you. Even more, it’ll allow you to have different projects built with various versions of Gradle.
Open your command line and move to the root directory of the starter project:
cd path/to/your/project/SocializifyStarter/
Executing gradlew Tasks
Execute the following command:
./gradlew tasks
You’ll see a list containing all available tasks:
> Task :tasks ------------------------------------------------------------ Tasks runnable from root project 'SocializifyStarter' ------------------------------------------------------------ Android tasks ------------- androidDependencies - Displays the Android dependencies of the project. ... Build tasks ----------- assemble - Assemble main outputs for all the variants. ... Build Setup tasks ----------------- init - Initializes a new Gradle build. ... Help tasks ---------- ... Install tasks ------------- ... Verification tasks ------------------ ... lint - Runs lint on the default variant. ... To see all tasks and more detail, run gradlew tasks --all To see more detail about a task, run gradlew help --task <task>
These commands exist to help you with tasks like project initialization, building, testing and analyzing. If you forget a specific command, just execute ./gradlew tasks
to refresh your memory.
gradlew assemble
Skim the list of commands again, and find commands starting with assemble under the Build tasks
section. Run the first command:
./gradlew assemble
Which will output something like:
BUILD SUCCESSFUL in 7s 74 actionable tasks: 74 executed
Verify the output by running the following command:
ls -R app/build/outputs/apk
The ls
command displays all files and directories in the specified directory. The -R
parameter forces this command to execute recursively. In other words, you’ll not only see the contents of the specified directory but also of child directories.
You’ll get the following output:
debug release
app/build/outputs/apk/debug:
app-debug.apk output-metadata.json
app/build/outputs/apk/release:
app-release-unsigned.apk output-metadata.json
Gradle generated both debug and release apks.
gradlew lint
Run the command:
./gradlew lint
The lint
command, and any commands that start with lint, analyzes the whole project looking for various mistakes, typos or vulnerabilities. The first command will find all the issues in a project with both critical and minor severity.
You’ll get the output with the link to a report with the results:
> Task :app:lintReportDebug Wrote HTML report to file:///path/to/project/SocializifyStarter/app/build/reports/lint-results-debug.html BUILD SUCCESSFUL in 2s 23 actionable tasks: 6 executed, 17 up-to-date
Review the report by typing the following on MacOS:
open app/build/reports/lint-results-debug.html
or on Linux:
xdg-open app/build/reports/lint-results-debug.html
The default browser on your computer will open with the specified file:
You can inspect all the issues found with code snippets and an expanded description of a possible solution. However, don’t focus too much on all of these issues – pay attention to the critical ones and fix them immediately. Minor issues shouldn’t necessarily warrant a refactoring, depending upon your team’s guidelines and processes.