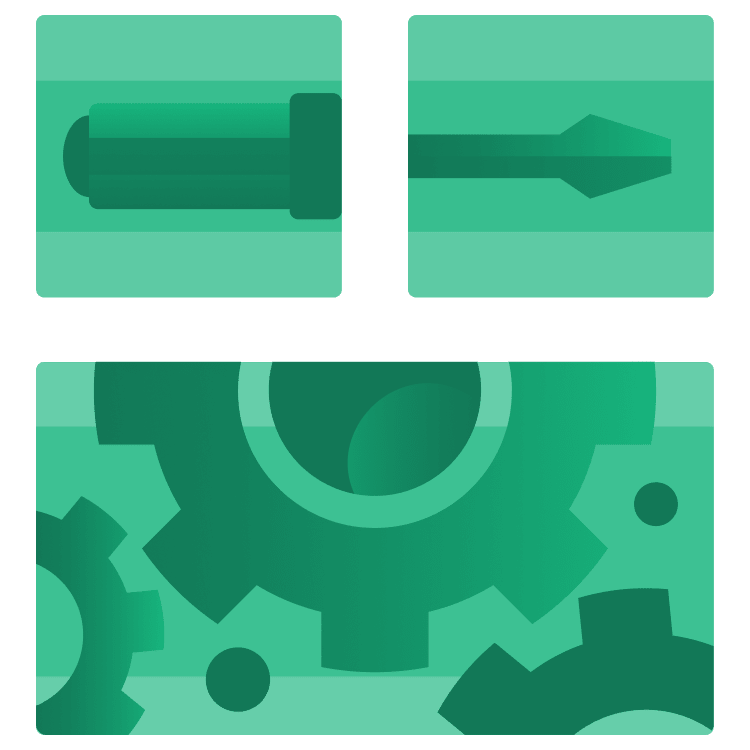
Introduction to Foundational Tools in Android
Learn about three of the most fundamental tools used in Android development in our new Foundational Tools for Android program. By Brian Moakley.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Introduction to Foundational Tools in Android
10 mins
Every task requires tools, whether building a new bookshelf or creating a new Android app. These tools are critical to the task at hand. Thankfully, with Android development, you don’t have to spend your life savings to acquire them. All these tools are free!
Android Studio, the Kotlin Programming Language, and Jetpack Compose are the three most essential tools you’ll use in your Android career. Of course, you can use many other tools to build your apps, but you’ll use these in every app.
Android Studio is an integrated developer environment that lets you write code, create emulators, and debug code as it runs. Kotlin is a modern object-oriented programming language that’s easy to write and understand. Jetpack Compose is a user interface toolkit that helps you lay out the various components of your apps, such as images and buttons.
In this article, you’ll dip your toe in Jetpack Compose. If you want to explore all these topics in depth, sign up for Kodeco’s Introduction to Foundational Tools in Android program. This program walks you through every step of the setup process, and you’ll even create a small app.
Create a Simple Android App
In this module, Fuad Kamal teaches you how to create a simple chat app in Jetpack Compose. In the process, you’ll:
Learn about composables. Composables are the building blocks of your user interface. You’ll learn how to define a composable and connect them like Lego bricks to design a killer user interface.
Understand some user interface components used to build a UI. You’ll learn to use buttons, rows, text, and other components in your Jetpack Compose app.
Combine composables into a singular composable. You’ll learn how to create custom composables that you can share throughout your app and even with others.
Seeing it in Action
The default Activity created for you contains the following function:
@Composable
fun Greeting(name: String, modifier: Modifier = Modifier) {
Text(
text = "Hello $name!",
modifier = modifier
)
}
There are two things of note in this code. First, it’s a function. Second, it’s annotated with @Composable. This is all you need to create a UI component in Compose, or, in Compose speak, a composable.
You might’ve noticed that the function name, Greeting(), is capitalized. Composable functions use Pascal case, unlike the camel case commonly used in Kotlin code. For example, a multi-word Compose function would be called ConversationContent instead of conversationContent. This distinction stems from composable functions returning UI objects, hence adopting the same naming convention as classes.
Greet Yourself
Update the value of Greeting() from “Android” to your name so that Kodeco Chat says hello to you.
You might immediately see the value change from “Android” to your name on the device or emulator without rerunning the app. If so, this is because of a Compose and Android Studio feature called Live Edit. If you don’t see it update live, run the app or enable Live Edit in Android Studio’s settings.
Build and run the app. When Android Studio finishes building and installing, Kodeco Chat will appear on your device or emulator:
Exploring Activities
A lifestyle of various activities — like cardio, strength training, and endurance — can keep you healthy. Although they’re all different, they each have a specific purpose or goal.
Android apps are similar — they’re built around a set of screens. Each screen is known as an Activity and built around a single task. For example, you might have a settings screen where users can adjust the app’s settings or a sign-in screen where users can log in with a username and password.
In the Project navigator on the left, ensure that the app folder is expanded. Navigate to MainActivity.kt in app/kotlin+java/com.kodeco.chat/MainActivity.kt.
Open the file, and you’ll see:
package com.kodeco.chat
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import com.kodeco.chat.ui.theme.KodecoChatTheme
// 1
class MainActivity : ComponentActivity() {
// 2
override fun onCreate(savedInstanceState: Bundle?) {
// 3
super.onCreate(savedInstanceState)
// 4
setContent {
KodecoChatTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Greeting("Fuad")
}
}
}
}
}
@Composable
fun Greeting(name: String, modifier: Modifier = Modifier) {
Text(
text = "Hello $name!",
modifier = modifier
)
}
@Preview(showBackground = true)
@Composable
fun GreetingPreview() {
KodecoChatTheme {
Greeting("Fuad")
}
}
MainActivity.kt is where the logic for your chat screen goes. Take a moment to explore what it does:
- MainActivity is declared as extending ComponentActivity. It’s your first and only Activity in this app. What ComponentActivity does isn’t important right now. All you need to know is that you must subclass to deal with content on the screen.
- onCreate() is the entry point to this Activity. It starts with the keyword override, meaning you’ll have to provide a custom implementation from the base ComponentActivity class.
- Calling the base’s implementation of onCreate() is not only important — it’s required. You do this by calling super.onCreate(). Android needs to set up a few things itself before your implementation executes, so you notify the base class that it can do so now.
- This line “composes” the given composable — everything that follows it in the braces {} — into the Activity. The content will become the root view of the Activity. The setContent{} block defines the Activity’s layout where composable functions are called. Composable functions can only be called from other composable functions. Hence, you see the Greeting inside onCreate() is just another function, defined below that, but it’s also marked with @Composable, which makes it a composable function.
Jetpack Compose uses a Kotlin compiler plugin to transform these composable functions into the app’s UI elements. For example, the Text composable function inside Greeting is, in turn, defined by the Compose UI library and displays a text label on the screen. You write composable functions to define a view layout that renders on your device screen.
Where to Go From Here?
Believe it or not, you’re just getting started with one of the four modules in the new Foundational Tools in Android course. By the end of it, you’ll build something that looks like this:
The course the following modules, which will take you from total beginner to a place where you’re comfortable with the fundamentals of Android programming:
- Introduction to Version Control
- Introduction to Android Studio
- Meet the Kotlin Programming Language
- Create a Simple Android App
You can experience this new content in one of two ways:
- The Foundational Tools in Android course is available now, and free for all Kodeco Personal and Kodeco for Business subscribers.
- If you prefer a holistic learning journey, guided by experienced mentors and culminating with a certificate of graduation, check out Kodeco’s brand-new Foundational Tools in Android on-demand bootcamp. You’ll be able to learn at your own pace, with the full support of an experienced Android developer, as you navigate engaging and challenging homework assignments that complement your learning of the subject materials.
This course is designed for total beginners, and provides a gentle on-ramp for people eager to start developing Android apps. Kodeco’s approach to learning allows you to code alongside the professionals with demos, and homework for on-demand bootcamps.
If you want to learn Android, there’s no better time!