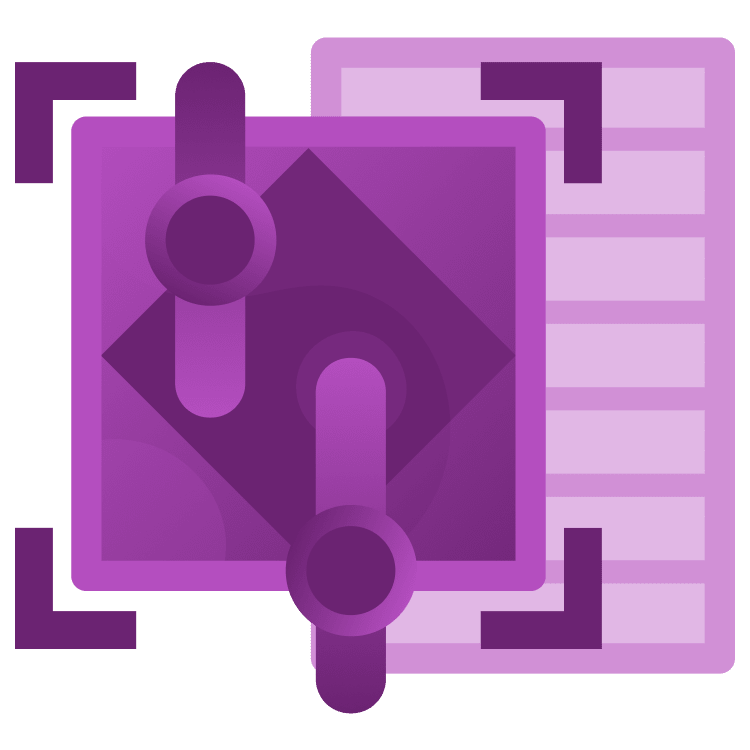
Getting Started with 2D Physics in Godot
Explore Godot’s physics engines to create dynamic games. Learn collision handling, add sound effects, and build engaging gameplay where luck and skill intersect! By Ken Lee.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Getting Started with 2D Physics in Godot
35 mins
- Get Started
- File Overview
- Main Scene
- Understanding 2D Physics Engine
- Understanding Different Physics Nodes
- Create a RigidBody2D node
- The Marble Scene
- About the PhysicsMaterial
- Friction and Rough
- Bounce and Absorbent
- Setting the PhysicsMaterial properties
- Gravity Setting
- Add the appearance
- Define the Collision Shape
- Spawning the Marble
- Creating the Marble Property
- Instantiate the Marble Node
- Limit the Spawn Location
- Adding the Score Logic
- Define the Score Triggers
- Handling the Score Signal
- Adding the StaticBody2D pegs
- Creating the Peg Scene
- Pegs Placement
- Setting Up the Snap Tool
- Position Pegs Using Snap Tools
- Fixing the Marble Stuck at the Peg
- Making a Complete Game Flow
- Marble Count Deduction
- Game Over Handing
- Sound and Background Music
- Adding Sound Effects for Pegs:
- Adding Background Music
- Where to Go From Here?
Game Over Handing
Now, you’ll implement the game over logic. It’s straightforward: check if all marbles have dropped in the score zone. If they have, display the game over dialog.
Add the following code to the _on_score_earned
function to check the game over condition.
func _on_score_earned(score):
total_score += score
$GUI.set_score(total_score)
# NEW CODE
dropped_count += 1
if(dropped_count >= MAX_MARBLE_COUNT):
$GameOverDialog.show_dialog(total_score)
The new code tracks the number of dropped marbles and displays the game over dialog when the dropped marble count is equal to or greater than the maximum marble count.
Now, run the game again and you’ll experience a complete game flow now.
When playing the game, you may notice that it’s not as engaging as desired. This could be due to its silence and lack of audio elements.
Sound and Background Music
Sound and music are essential elements in game development. Sound effects enhance feedback to the player for their actions, while background music enhances the atmosphere of the game. Both elements contribute to making a game more engaging and immersive.
Adding Sound Effects for Pegs:
Now, you’ll add a sound effect to the peg collision. Open the peg.tscn scene and add a new ChildNode called AudioStreamPlayer2D.
Then, drag the sound file sounds/marble-hit.mp3 from the FileSystem dock to the AudioStreamPlayer2D node you’ve just created.
On the script side, you need to create a custom class for the peg node and add a method to play the sound effect.
First, create a new script for the Peg node.
Right-click the Peg node in the Scene dock and select New Script. Change the path to res://scripts/peg.gdd and click Create.
After that, switch to the script view and add the following code in the peg.gd script:
extends StaticBody2D
class_name Peg
func play_sound():
$AudioStreamPlayer2D.play()
The above code creates a new custom class called Peg with a public method play_sound
to play the marble hit sound. In the marble logic, modify the collision handler, _on_body_entered
, to play the hit sound of the peg.
Open the marble.gd file and modify the _on_body_entered
method as follows:
func _on_body_entered(body):
_add_random_force()
# Trigger Hit Sound Effect
if body is Peg:
body.play_sound()
The new code checks if the marble collides with the Peg node. If so, it calls the play_sound
method to play the sound effect.
Now, run the game and you’ll hear the sound effect you’ve just added.
Adding Background Music
Now, you’ll add background music to create a relaxed atmosphere for players.
Open the main_scene.tscn
in the FileSystem dock. Once the scene is open, select the MainScene node.
Right-click and select Add Child Node in the menu. Then, choose AudioStreamPlayer2D to create the node.
When the node is created, rename it to BGMPlayer for clarity.
Next, drag the background music file music/bgm.mp3 from the FileSystem dock to the Stream property in the Inspector view of the BGMPlayer node.
Unlike the sound effect, the background music should loop forever and start playing automatically when the scene loads. However, the Loop property is disabled and the Autoplay property is turned off by default.
To enable the Loop property, click the small dropdown arrow next to the Stream property to reveal additional options.
Select Make Unique from the dropdown menu to ensure the resource is only shared to this particular instance.
After that, the Loop property will be enabled. Now, turn the Loop property ON to make the music play forever.
To turn on the Autoplay property, locate the Autoplay property in the Inspector view of the BGMPlayer node. Then, toggle it to ON to make the background music start playing automatically when the scene loads.
These are the properties after setting up:
Now, run the game to check if the background music is playing.
You may notice the game is much better than the muted version, thanks to the sound effects and background music you added.
Where to Go From Here?
You now know the basic usage of the Godot 2D physics engine, and you can use this knowledge to develop your own physics-based games.
You can also customize the game by changing the marble size, altering the pattern, and adding more pegs to the game board.
Additionally, you can enhance your game by adding more visual and sound effects, such as effects when the player scores.
For more in-depth information, check out the Physics section in Godot’s official documentation.
If you have any questions or comments, please join the discussion below.