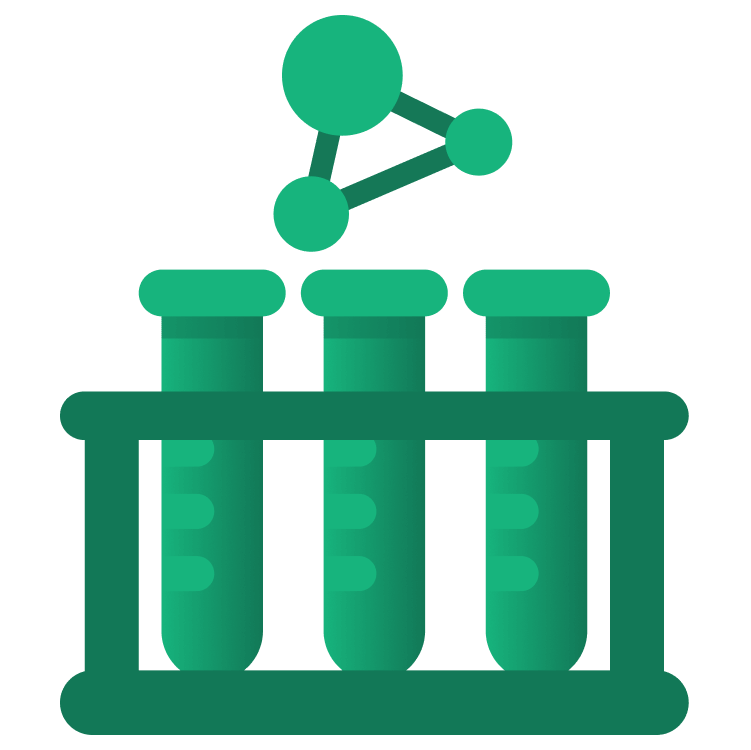
Test-Driven Development in Android
In this course, you are going to learn how to create scalable and maintainable apps by applying several testing methodologies like the Red-Green-Refactor steps and frameworks such as JUnit and Mockito. You will also learn about SOLID principles, design patterns and the best architectures for testing. By Aldo Olivares.
Who is this for?
This course is intended for intermediate and advanced level Android Developers that have a good background in Android Development, Kotlin and Software Development. Experience with testing libraries such as Mockito and JUnit is recommended but not required.
Covered concepts
- Android App Architectures
- Red-Green-Refactoring
- The Testing Pyramid
- Mockito & Junit
- Espresso
- Testing the Network layer
- Testing the Persistance layer
- Testing the UI
Part 1: Unit Tests
In this episode you are going to learn what test-driven development is and its advantages over traditional development practices.
A very important part of test-driven development are the Red-Green-Refactor steps. In this episode you will learn what they are and how to use them regardless of the development platform you are using.
In this episode I am going to give you a short introduction to Software Testing and its importance.
JUnit is a powerful java framework that allows you to perform unit testing in your Android project. In this episode you are going to set up JUnit and create your first unit test.
In this lesson we are going to create our first unit tests with TDD using JUnit.
Continuing from the previous episode, we are going to create more unit tests using JUnit.
Tired of writing the same code in different tests? In this episode you are going to learn how to use annotations to execute code automatically before each test is run.
In this episode you’re going to test your Answer and Question classes with Mockito and JUnit.
The last step of the Red-Green-Refactor steps is to, well, refactor your code. This time you will refactor the code of your cocktail app and verify that it is still working as expected by executing all of your tests at once.
Part 2: Integration Tests
A very important part of test-driven development are the Red-Green-Refactor steps. In this episode you will learn what they are and how to use them regardless of the development platform you are using.
Here we are going to create a test class for all of our integration tests.
Now that you have reviewed the app and the project structure for this section you’re ready to create the first integration test for your project. In this episode you’ll create your first integration test.
Just like integration tests, you’ll need practice to master integration tests. In this episode you’ll create even more integration tests for your View Models.
We just need to add one more test before wrapping up this section of the course. In this episode we will add one more integration test to our app before refactoring our code.
As a final step for this section, we’re going to refactor our code and make sure that everything still works as expected by executing your tests.
Part 3: Test-Driven Development: UI Tests
Normally, when creating new Android Projects Espresso dependencies are already added for you since it is a very popular library. Still, we need to make sure everything works fine before working with it. In this episode we are are going to verify that espresso dependencies are added and create our test class.
In this episode we will create a UI test by automatically lauching an Activity and verifying if a button is displayed on screen.
Now that you know how to verify if a button is visible on the screen you’ll learn how to check if a certain text is displayed. This is specially useful for alerts and messages you want to display.
When a user taps the New Joke button a new joke should appear on our screen. In our final episode we are going to write the most complex test by creating a Joke, a list of answers and verifying it is displayed on our screen.