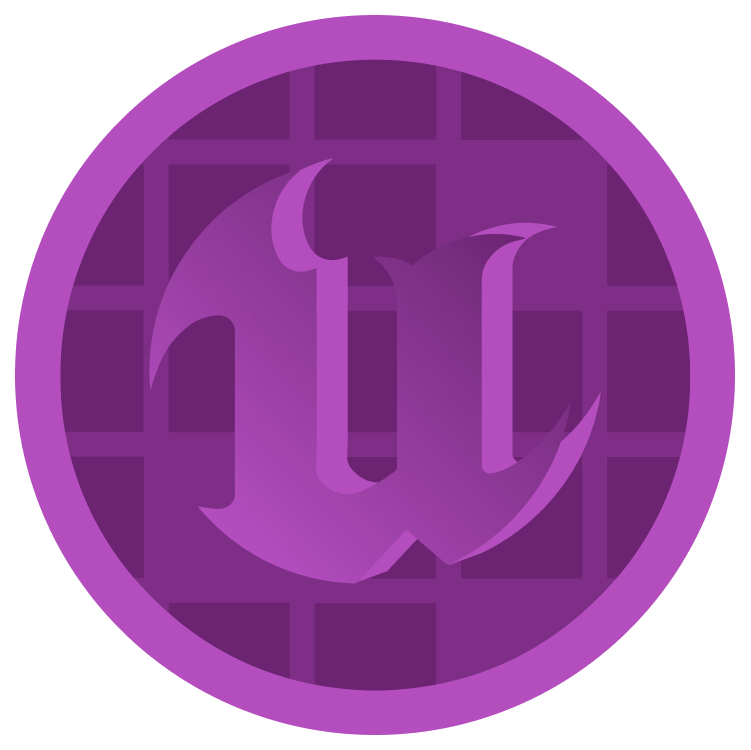
Unreal Engine 5 UI Tutorial
In this Unreal Engine 5 UI tutorial, you’ll learn how to create, display and update a HUD. By Ricardo Santos.
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Sign up/Sign in
With a free Kodeco account you can download source code, track your progress, bookmark, personalise your learner profile and more!
Create accountAlready a member of Kodeco? Sign in
Contents
Unreal Engine 5 UI Tutorial
25 mins
- Getting Started
- Using Widgets
- Creating a Widget
- The UMG UI Designer
- Creating a Text Widget
- Creating an Image Widget
- Using Anchors
- Creating the Timer
- Displaying the HUD
- Storing References
- Creating the Variable
- Setting the Reference
- Functions
- Organizing
- Reusability
- Updating a Widget
- Creating the Update Function
- Calling the Update Function
- Bindings
- Creating a Binding
- Where to Go From Here?
Creating the Variable
Open WBP_HUD and switch to Graph mode by going to the Editor Mode and selecting Graph.
You’ll notice that the Palette panel was replaced by the My Blueprint tab. Navigate to it and create a new variable called GameManager.
Go to the Details panel and click the drop-down next to Variable Type. Search for BP_GameManager and select BP Game Manager\Object Reference.
Setting the Reference
Click Compile and then open BP_GameManager.
Locate the Create Widget node and then left-click and drag the Return Value pin. Release left-click on an empty space and then select Set Game Manager from the menu.
Afterward, link the Add to Viewport node to the Set Game Manager node.
Next, create a Self node and connect it to the left pin of the Set Game Manager node. The Self node will be listed as Get a reference to self.
Now, when WBP_HUD is created, it will have a reference to BP_GameManager.
In the next section, you’ll learn how to update a widget with the help of functions.
Functions
In Blueprints, functions are graphs similar to the Event Graph. Unlike the Event Graph, you can call a function using a node. But why would you want to do this? Read on to find out!
Organizing
One of the reasons to use functions is organization. By using functions, you can collapse multiple nodes into a single node.
Take a look at the Event BeginPlay section of BP_GameManager. There are two functions: Restart and SetUpCamera.
Here’s what that section would look like without functions:
As you can see, it looks a lot cleaner using functions.
Reusability
Another reason to use functions is reusability. For example, if you wanted to reset the counter and timer, you could easily do so using the Restart function.
This saves you the work of having to recreate the nodes every time you want to reset those variables.
Now that you know what functions are, you’ll use one to update the CounterText widget.
Updating a Widget
By default, Text widgets are not accessible from Blueprints. This means you won’t be able to set their Text property. Luckily, this is an easy fix.
Click Compile and then open WBP_HUD. Switch to Designer mode.
Select CounterText and then go to the Details panel. Check the Is Variable checkbox located at the very top.
Now, you’ll be able to update CounterText. The next step is to create a function to update the text.
Creating the Update Function
Switch back to Graph mode and then go to the My Blueprint tab. Click the + sign to the right of the Functions section.
This will create a new function and take you to its graph. Rename the function to UpdateCounterText.
By default, the graph will contain an Entry node. When the function executes, this is where it will start.
To make CounterText display the ShapesCollected variable, you’ll need to link them.
Drag the GameManager variable into the graph. Left-click and drag its pin and then release left-click on an empty space. Select Get Shapes Collected from the menu.
To set the text, you’ll use the SetText (Text) node. Drag the CounterText variable into the graph. Left-click and drag its pin and then release left-click on an empty space. From the menu, add a SetText (Text) node.
The SetText (Text) only accepts an input of type Text. However, the ShapesCollected variable is of type Integer. Luckily, Unreal will do the conversion automatically when you try to plug an Integer into a Text input.
Connect the ShapesCollected variable to the In Text pin for the Set Text (Text) node. Unreal will automatically create a ToText (int) node for you.
To complete the function, connect the Entry node to the Set Text (Text) node.
Order of events:
- When you call UpdateCounterText, the function will get the ShapesCollected variable from BP_GameManager.
- The ToText (int) node converts the value of ShapesCollected to a Text type.
- SetText (Text) will set the text for CounterText to the value from ToText (int).
Next, you have to call UpdateCounterText whenever the player collects a shape.
Calling the Update Function
The best place to call UpdateCounterText is right after the game increments ShapesCollected. I’ve created a function called IncrementShapesCollected that increments the counter for you. The shapes call this function whenever they overlap the player.
Click Compile and then go back to BP_GameManager.
Before you can call UpdateCounterText, you need a reference to WBP_HUD. See if you can store a reference by yourself first. If you get stuck, read on!
- Locate the section where you created and displayed the WBP_HUD.
- Left-click and drag the Return Value pin of the Create Widget node.
- Release left-click on an empty space and then select Promote to variable from the menu.
- Add the new node to the end of the node chain.
Once you have created the variable, rename it to HUDWidget.
Next, drag-click the right pin of the Set HUDWidget node and release on an empty space. Add an UpdateCounterText node. This will make sure CounterText displays the value of ShapesCollected when the game starts.
Afterward, navigate to the My Blueprint panel and go to the Functions section. Double-click on IncrementShapesCollected to open its graph.
Drag the HUDWidget variable into the graph. Left-click and drag its pin and release on an empty space. Add an UpdateCounterText node and connect it like so:
Now, whenever IncrementShapesCollected executes, it will increment ShapesCollected and then call UpdateCounterText. This function will then update CounterText to the value of ShapesCollected.
Click Compile and then close BP_GameManager. Press Play and collect some shapes to see the CounterText widget update.
In the next section, you’ll update the TimerText widget using a different method called binding.
Bindings
Bindings allow you to update certain widget properties automatically. The property must have the Bind drop-down to be bindable.
You can bind properties to a function or variable contained within the widget. The binding will constantly obtain a value from the function or variable. It will then set the bound property to that value.
You might be wondering why you shouldn’t just use bindings all the time. Bindings are inefficient because they are constantly updating. This means the game wastes time updating the property even if there isn’t any new information. Compare it to the previous method, where the widget only updates when needed.
That said, bindings are great for frequently changing elements, such as the timer. Let’s go ahead and create a binding for TimerText.